Breadth-First Search(BFS)
Intruction
BFS stands for Breadth-First Search, which is a graph search algorithm that starts from a given node and explores the graph by expanding the nodes at the current depth before moving on to the next depth. BFS explores the graph branch by branch, trying to find the target node as quickly as possible.
The main advantage of BFS is that the shortest path to the target node is found when the target node is first visited. This is because BFS guarantees that all paths to the target node have been explored by the time it is first visited.
BFS is typically implemented using a queue, where each iteration of the algorithm consists of removing a node from the queue and adding its unvisited neighbors to the queue. This process continues until the target node is found or the queue is empty.
BFS is useful for solving problems such as finding the shortest path between two nodes, determining the order in which nodes should be processed, and identifying cycles in a graph.
Example:
542. 01 Matrix
Given an m x n binary matrix mat, return the distance of the nearest 0 for each cell.
The distance between two adjacent cells is 1.
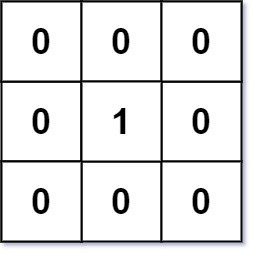
Input: mat = [[0,0,0],[0,1,0],[0,0,0]]
Output: [[0,0,0],[0,1,0],[0,0,0]]
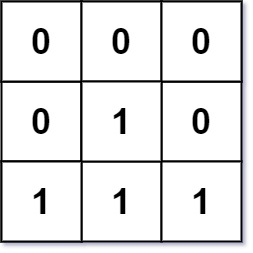
Input: mat = [[0,0,0],[0,1,0],[1,1,1]] Output: [[0,0,0],[0,1,0],[1,2,1]]
Solution
1 | class Solution: |